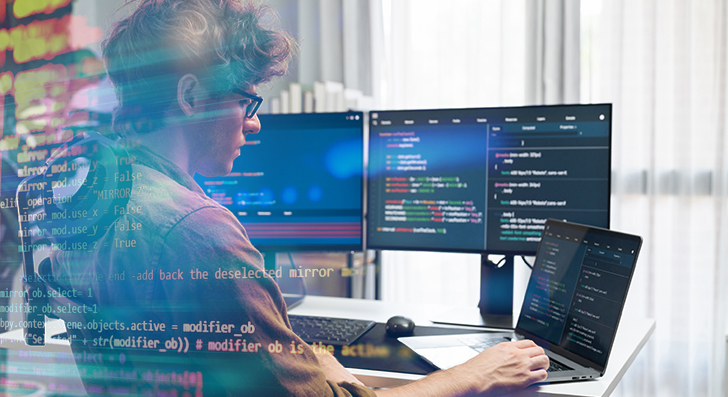
Scalability suggests your application can handle advancement—additional end users, much more data, and a lot more site visitors—with out breaking. As a developer, making with scalability in mind will save time and pressure later. Below’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it should be section of the plan from the start. Many apps fail whenever they develop fast mainly because the original layout can’t handle the extra load. To be a developer, you should Imagine early about how your program will behave under pressure.
Start off by creating your architecture to get flexible. Keep away from monolithic codebases where by every little thing is tightly related. Rather, use modular structure or microservices. These patterns split your application into smaller, impartial pieces. Every module or assistance can scale By itself without having affecting The entire procedure.
Also, consider your database from working day one. Will it want to manage one million buyers or maybe 100? Pick the right kind—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t want them nonetheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t produce code that only is effective less than current circumstances. Take into consideration what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the databases slow down?
Use design and style designs that help scaling, like message queues or celebration-driven techniques. These aid your app deal with much more requests with out obtaining overloaded.
When you build with scalability in mind, you're not just making ready for achievement—you happen to be lowering long term headaches. A well-prepared technique is simpler to keep up, adapt, and expand. It’s much better to arrange early than to rebuild afterwards.
Use the best Database
Choosing the suitable database is really a crucial part of setting up scalable apps. Not all databases are developed the same, and utilizing the Mistaken one can gradual you down and even cause failures as your application grows.
Start out by knowing your data. Could it be extremely structured, like rows inside of a desk? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are sturdy with relationships, transactions, and regularity. They also guidance scaling methods like browse replicas, indexing, and partitioning to deal with extra targeted traffic and data.
In the event your knowledge is a lot more versatile—like person activity logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing substantial volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your go through and produce patterns. Do you think you're accomplishing plenty of reads with much less writes? Use caching and read replicas. Do you think you're managing a hefty compose load? Check into databases that can manage significant write throughput, and even celebration-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Assume in advance. You might not need Superior scaling characteristics now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your details depending on your access designs. And constantly watch databases effectiveness when you improve.
To put it briefly, the ideal databases will depend on your application’s framework, velocity requires, And exactly how you be expecting it to improve. Acquire time to choose correctly—it’ll preserve plenty of problems later.
Improve Code and Queries
Speedy code is key to scalability. As your application grows, just about every smaller delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop effective logic from the start.
Get started by producing clear, straightforward code. Avoid repeating logic and take away everything needless. Don’t choose the most elaborate Resolution if a simple a single works. Keep the features short, centered, and easy to check. Use profiling equipment to locate bottlenecks—sites the place your code requires as well extensive to run or uses an excessive amount memory.
Subsequent, evaluate your database queries. These normally sluggish things down a lot more than the code itself. Be sure Every single question only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And steer clear of executing a lot of joins, In particular across huge tables.
For those who discover the exact same data getting asked for again and again, use caching. Retailer the final results temporarily making use of applications like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to take a look at with significant datasets. Code and queries that function fantastic with one hundred data could crash every time they have to handle 1 million.
In brief, scalable apps are rapidly applications. Maintain your code limited, your queries lean, and use caching when needed. These actions aid your application remain easy and responsive, whilst the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more consumers and a lot more targeted visitors. If everything goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your app quick, stable, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server accomplishing the many operate, the load balancer routes end users to distinct servers according to availability. This suggests no one server will get overloaded. If 1 server goes down, the load balancer can send traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it might be reused swiftly. When customers ask for precisely the read more same info all over again—like a product webpage or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it through the cache.
There are two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
two. Customer-side caching (like browser caching or CDN caching) outlets static information near the user.
Caching lowers database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust usually. And normally ensure your cache is up-to-date when information does adjust.
In short, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app take care of extra customers, keep speedy, and Get well from complications. If you plan to expand, you require both.
Use Cloud and Container Equipment
To develop scalable purposes, you need resources that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They offer you flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t should obtain components or guess upcoming potential. When visitors raises, you are able to insert additional means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also present products and services like managed databases, storage, load balancing, and stability instruments. You may center on constructing your app rather than handling infrastructure.
Containers are One more crucial Instrument. A container packages your application and almost everything it should run—code, libraries, configurations—into just one device. This makes it easy to maneuver your app in between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred tool for this.
Once your app utilizes various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your application into companies. You are able to update or scale components independently, which happens to be great for general performance and dependability.
To put it briefly, employing cloud and container tools suggests you are able to scale rapid, deploy very easily, and Get better rapidly when complications occur. If you prefer your app to increase without boundaries, get started working with these tools early. They preserve time, cut down threat, and make it easier to stay focused on making, not fixing.
Check Anything
In the event you don’t keep an eye on your software, you received’t know when issues go Mistaken. Checking helps you see how your app is doing, location concerns early, and make superior conclusions as your application grows. It’s a important Portion of making scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app too. Control just how long it will require for people to load web pages, how frequently glitches transpire, and wherever they come about. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or perhaps a service goes down, you should get notified immediately. This assists you fix issues speedy, generally in advance of end users even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and facts boost. Without having checking, you’ll miss out on signs of hassle right up until it’s as well late. But with the ideal equipment set up, you keep on top of things.
In a nutshell, monitoring will help you keep your application dependable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t just for significant organizations. Even tiny applications require a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you could Construct applications that grow easily without the need of breaking under pressure. Start off compact, Believe major, and build wise.